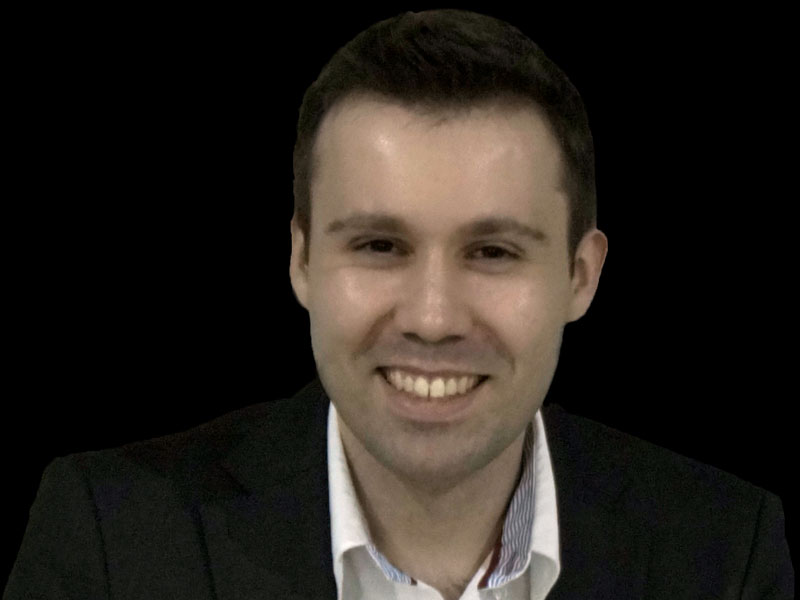
Licenciado en Periodismo y en Economía, con Máster en Márketing Digital y Máster de SEO avanzado con BIG SEO, actualmente soy SEO Manager en Agencia con más de 4 años de experiencia.
He trabajado en empresas internacionales en España y en México, en medios de comunicación digitales, y dirigido mis propios proyectos en estos y como nichero. [Casos de éxito SEO]